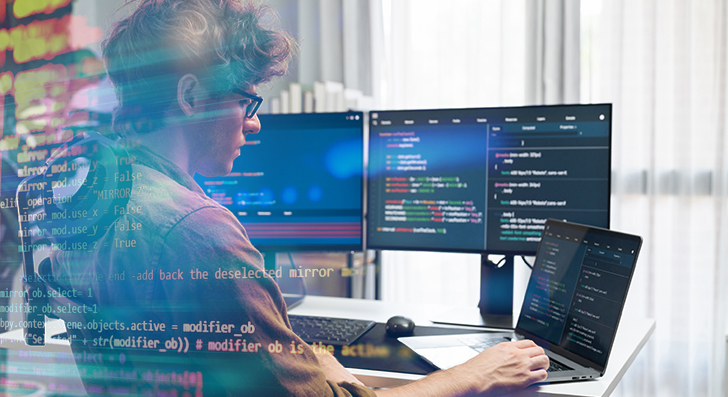
Scalability implies your software can cope with progress—a lot more customers, extra facts, and a lot more targeted traffic—without having breaking. As a developer, making with scalability in mind will save time and anxiety later. Below’s a clear and simple guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few applications are unsuccessful whenever they develop speedy due to the fact the original structure can’t manage the additional load. Like a developer, you might want to Feel early regarding how your method will behave stressed.
Start by planning your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly related. Rather, use modular style and design or microservices. These patterns break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having impacting The complete system.
Also, think of your databases from working day one. Will it require to manage one million users or perhaps a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t need them yet.
An additional critical position is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day conditions. Consider what would take place Should your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use structure styles that aid scaling, like information queues or celebration-pushed programs. These support your app deal with much more requests with out acquiring overloaded.
Once you Construct with scalability in mind, you are not just making ready for achievement—you are lowering long term headaches. A perfectly-prepared program is easier to maintain, adapt, and mature. It’s superior to organize early than to rebuild later.
Use the Right Databases
Selecting the correct databases can be a crucial A part of setting up scalable apps. Not all databases are developed exactly the same, and utilizing the Mistaken one can gradual you down and even trigger failures as your application grows.
Commence by understanding your facts. Could it be highly structured, like rows in a very table? If Certainly, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are potent with associations, transactions, and consistency. Additionally they assistance scaling approaches like go through replicas, indexing, and partitioning to take care of far more traffic and facts.
Should your details is much more adaptable—like user exercise logs, item catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more effortlessly.
Also, take into consideration your study and produce patterns. Do you think you're doing a great deal of reads with much less writes? Use caching and read replicas. Will you be managing a hefty write load? Investigate databases which can take care of superior create throughput, or maybe event-primarily based knowledge storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume ahead. You may not will need Sophisticated scaling functions now, but picking a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Keep away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And always monitor database overall performance as you expand.
In brief, the correct database is determined by your app’s construction, speed wants, And the way you count on it to develop. Consider time to pick sensibly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Fast code is essential to scalability. As your application grows, just about every smaller delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated Answer if a straightforward just one performs. Keep your capabilities quick, focused, and straightforward to test. Use profiling applications to search out bottlenecks—areas where your code can take way too long to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These generally slow matters down over the code itself. Be sure Every question only asks for the data you really need. Keep away from SELECT *, which fetches anything, and rather decide on specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, Particularly throughout significant tables.
Should you see a similar information currently being asked for again and again, use caching. Keep the results briefly using equipment like Redis or Memcached so you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more successful.
Make sure to examination with significant datasets. Code and queries that function good with one hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These actions aid your application remain smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers plus more traffic. If everything goes via 1 server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching are available in. These two tools help keep the application rapid, steady, and scalable.
Load balancing spreads incoming traffic throughout various servers. In lieu of a person server executing the many get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Instruments like Nginx, HAProxy, or cloud-based mostly answers from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for precisely the same details again—like an item web page or a profile—you don’t should fetch it with the database when. You may serve it within the cache.
There are 2 popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lessens database load, increases speed, and can make your app far more efficient.
Use caching for things that don’t improve usually. And always ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective applications. With each other, they help your application deal with additional buyers, stay rapidly, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to purchase hardware or guess potential capability. When targeted traffic boosts, you may increase extra resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on building your application in place of taking care of infrastructure.
Containers are A different critical Device. A container deals your app and all the things it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Device for this.
Whenever your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy easily, and Get well quickly when challenges occur. In order for you your app to increase without the need of limitations, get started utilizing these instruments early. They save time, lessen hazard, and enable you to keep centered on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you received’t know when things go Improper. Checking can help you see how your app is executing, location challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just watch your servers—watch your application as well. Keep watch over how long it requires for end users to load webpages, how often problems come about, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for critical difficulties. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you deal with difficulties rapidly, typically just before customers even notice.
Checking is likewise valuable once you make adjustments. In the event you deploy a completely new element and see a spike in errors or slowdowns, you can roll it again just before it leads to serious problems.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be on top of things.
In brief, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works properly, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need to here have a strong foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Make apps that increase effortlessly with out breaking stressed. Get started little, Consider big, and Construct clever.